Navigation is an essential element for a website which makes it easier for the user to go through the content in the different pages. If you have lots of pages on your website, you must include pagination on your pages.
CSS pagination is one of the most convenient ways to index different pages to the homepage of the website. You can also include animation and different styles to show your creativity while developing. That way, you could also be able to enhance the experience of your users.
Here you are going to learn about some of the most creative styles of pagination coding, which you can use to design engaging pages on your website. You can go through all of them and opt for the best one to improve the navigation on your website.
What Is Pagination?
It is a process of dividing content in the form of discrete pages. While in coding language, we can use pagination to assign consecutive numbers to different webpages. Each number represent the different link of the webpage, which makes it easier for the viewer to jump to any webpage with a single click.
Here we have an example of basic pagination:
<!DOCTYPE html>
<html>
<head>
<style>
.pagination { display: inline-block;
padding: 0;
margin: 0;
}
.pagination li {display: inline;}
.pagination li a { color: red;
float: left;
padding: 8px 16px;
text-decoration: none;
}
</style>
</head>
<body>
<h2>Basic Pagination</h2>
<div class="pagination">
<li><a href="#">1</a></li>
<li><a class="active" href="#">2</a></li>
<li><a href="#">3</a></li>
<li><a href="#">4</a></li>
<li><a href="#">5</a></li>
<li><a href="#">6</a></li>
<li><a href="#">7</a></li>
</div>
</body>
</html>
Why Use Pagination:-
It is one of the best ways to spread out content and keywords to the different pages on your website. With more pages, you have enough space to add more content on your website. Even if you want to separate a long content, pagination can be pretty handy.
Pagination also plays an important role in the SEO of the website. It makes it easier for the machine learning of the search engines to index the content of the website and rank them accordingly.
Here are shown some of the paginations which you can implement on your website.
Hoverable Pagination:-
It gives the animation effect to the navigation of web pages. The highlighted number shows the selected page using .active class. While you can use :hover to change the selection of pages when the cursor passes over them. When a user clicks on a number that number would become active and it would get highlighted on the screen.
<html> <head> <style> .pagination { display: inline-block; } .pagination a { color: black; float: left; padding: 8px 16px; text-decoration: none; } .pagination a.active { background-color: #4CAF50; color: white; } .pagination a:hover:not(.active) {background-color: #ddd;} </style> </head> <body> <h2>Hoverable Pagination</h2> <p>Move the cursor over the numbers.</p> <div class="pagination"> <a href="#">«</a> <a href="#">1</a> <a class="active" href="#">2</a> <a href="#">3</a> <a href="#">4</a> <a href="#">5</a> <a href="#">6</a> <a href="#">»</a> </div> </body> </html>
![]()
Bordered Pagination:
Nowadays, most of the web developers opt for bordered pagination. As it seems more responsive and by using different colours, you can also enhance the look of your website. In this type of pagination, block shape border covers each number. Based on the movement of the cursor on the bordered area, numbers got highlighted. Have a look at this code:
<html>
<head>
<style>
ul.pagination {
display: inline-block;
padding: 0;
margin: 0;
}
ul.pagination li {display: inline;}
ul.pagination li a {
color: black;
float: left;
padding: 8px 16px;
text-decoration: none;
border: 1px solid black;
}
ul.pagination li a.active {
background-color: blue;
color: white;
border: 1px solid grey;
}
ul.pagination li a:hover:not(.active) {background-color: lightblue;}
</style>
</head>
<body>
<h2>Bordered Pagination</h2>
<ul class="pagination">
<li><a href="#">?</a></li>
<li><a href="#">1</a></li>
<li><a class="active" href="#">2</a></li>
<li><a href="#">3</a></li>
<li><a href="#">4</a></li>
<li><a href="#">5</a></li>
<li><a href="#">6</a></li>
<li><a href="#">7</a></li>
<li><a href="#">?</a></li>
</ul>
</body>
</html>
Pagination Size:
It is a type of responsive pagination where the bordered number changes its font size when the cursor passes over them. The selected page number is the active block selected, which shows in a different colour. The change in the font size seems more noticeable to the viewers. That is the reason why some of the developers use this type of pagination on their website.
<html>
<head>
<style>
ul.pagination { display: inline-block;
padding: 0;
margin: 0;
}
ul.pagination li {display: inline;
}
ul.pagination li a { color: black;
float: left;
padding: 8px 16px;
text-decoration: none;
border: 1px solid black;
font-size: 22px;
}
ul.pagination li a.active { background-color: brown;
color: white;
border: 1px solid grey;
}
ul.pagination li a:hover:not(.active) {font-size: 32px;}
</style>
</head>
<body>
<h2>Pagination Size</h2>
<p>Change the font-size property to make the pagination smaller or bigger.</p>
<ul class="pagination">
<li><a href="#">?</a></li>
<li><a href="#">1</a></li>
<li><a class="active"href="#">2</a></li>
<li><a href="#">3</a></li>
<li><a href="#">4</a></li>
<li><a href="#">5</a></li>
<li><a href="#">6</a></li>
<li><a href="#">7</a></li>
<li><a href="#">?</a></li>
</ul>
</body>
</html>

Centred Pagination:
Usually, pagination takes the left side alignment. However, if you want to move it to the centre of the page, you can use centred pagination coding. Most of the coding part is similar to other responsive pagination. While you need to add the alignment code to align the pagination at the centre of the webpage.
<!DOCTYPE html>
<html>
<head>
<style>
ul.pagination { display: inline-block;
padding: 0;
margin: 0;
}
ul.pagination li {display: inline;}
ul.pagination li a { color: black;
float: left;
padding: 8px 16px;
text-decoration: none;
border: 1px solid #ddd;
}
ul.pagination li a.active { background-color: blue;
color: white;
border: 1px solid black;
}
ul.pagination li a:hover:not(.active) {background-color: lightblue;}
div.center {text-align: center;}
</style>
</head>
<body>
<h2>Centered Pagination</h2>
<div class="center">
<ul class="pagination">
<li><a href="#">?</a></li>
<li><a href="#">1</a></li>
<li><a class="active" href="#">2</a></li>
<li><a href="#">3</a></li>
<li><a href="#">4</a></li>
<li><a href="#">5</a></li>
<li><a href="#">6</a></li>
<li><a href="#">7</a></li>
<li><a href="#">?</a></li>
</ul>
</div>
</body>
</html>
Breadcrumb Pagination:
If you want to include a few pages on your main website pages, you can make use of breadcrumb pagination. Most of the website make use of breadcrumb pagination at the top of the screen. You can use this pagination to differentiate the contents on the pages based on the categories.
<!DOCTYPE html>
<html>
<head>
<style>
ul.breadcrumb { padding: 8px 16px;
list-style: none;
background-color: #da614e;
}
ul.breadcrumb li {display: inline;}
ul.breadcrumb li+li:before { padding: 8px;
color: green;
content: "/\00a0";
}
ul.breadcrumb li a {color: white;}
</style>
</head>
<body>
<h2>MyPage</h2>
<ul class="breadcrumb">
<li><a href="#">Home</a></li>
<li><a href="#">About us</a></li>
<li><a href="#">Features</a></li>
<li><a href="#">Pricing</a></li>
</ul>
<p><strong>Note:</strong>Breadcrumb pagination.</p>
</body>
</html>
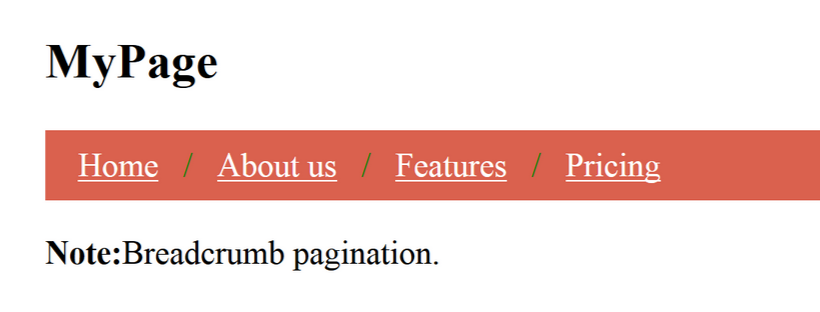
Also Read:
How To Start Building Your Own Cloud Based SaaS Product?
Conclusion:
These are some of the coolest examples of pagination which you can adept in your website. You can include Javascript ( jQuery ) in your CSS coding to make your pagination more interesting. If you want to share your ideas regarding pagination, please feel free to write to me in the comment section below.
Recent comments